SM2是由中國國家密碼管理局制定的公鑰密碼算法,屬于國家密碼標準之一。SM2算法基于橢圓曲線密碼學,兼具安全性和高效性,被廣泛應用在金融、電子政務和電子商務等領域。
特點
安全性:SM2基于橢圓曲線密碼學,提供高強度的安全保障。
高效性:在相同的安全強度下,SM2比RSA和DSA更加高效,計算復雜度較低。
國家標準:SM2是中國國家密碼標準GB/T 32918,具有國家認可的權威性。
應用場景
數字簽名:用于身份驗證和不可否認性。
數據加密:用于保護敏感信息的傳輸與存儲。
密鑰交換:用于安全的密鑰分發和交換過程。
代碼示例
第三方庫BouncyCastle
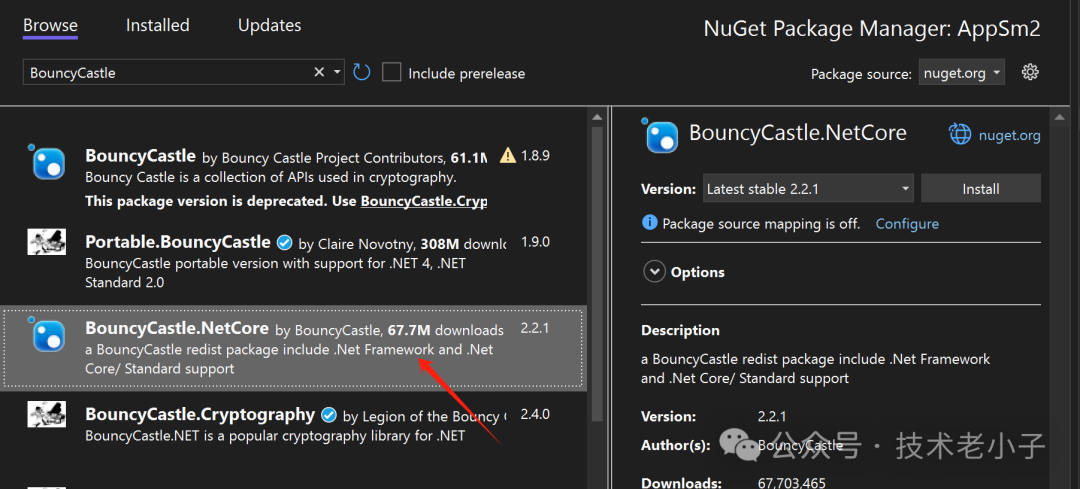
using Org.BouncyCastle.Asn1.X9;
using Org.BouncyCastle.Crypto.Generators;
using Org.BouncyCastle.Crypto;
using Org.BouncyCastle.Crypto.Parameters;
using Org.BouncyCastle.Security;
using Org.BouncyCastle.Asn1.Sec;
using Org.BouncyCastle.Asn1.GM;
using System.Security.Cryptography.X509Certificates;
using Org.BouncyCastle.Crypto.Engines;
using Org.BouncyCastle.Math;
namespace AppSm2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnCreateKey_Click(object sender, EventArgs e)
{
// 1. 生成SM2密鑰對
var keyPair = GenerateSM2KeyPair();
var publicKey = (ECPublicKeyParameters)keyPair.Public;
var privateKey = (ECPrivateKeyParameters)keyPair.Private;
File.WriteAllText("./public.dat", Convert.ToBase64String(publicKey.Q.GetEncoded()));
File.WriteAllText("./private.dat", Convert.ToBase64String(privateKey.D.ToByteArrayUnsigned()));
}
/// <summary>
/// 生成公私鑰
/// </summary>
/// <returns></returns>
private AsymmetricCipherKeyPair GenerateSM2KeyPair()
{
// 使用國標曲線參數 "Wapi" 被GMNamedCurves定義 (SM2標準曲線)
X9ECParameters sm2Params = GMNamedCurves.GetByName("sm2p256v1");
var ecParams = new ECDomainParameters(sm2Params.Curve, sm2Params.G, sm2Params.N, sm2Params.H);
var keyGenerationParameters = new ECKeyGenerationParameters(ecParams, new SecureRandom());
var keyPairGenerator = new ECKeyPairGenerator();
keyPairGenerator.Init(keyGenerationParameters);
return keyPairGenerator.GenerateKeyPair();
}
private void btnEncrypt_Click(object sender, EventArgs e)
{
// 將Base64格式公鑰轉換回ECPublicKeyParameters
var restoredPublicKey = RestorePublicKeyFromBase64(File.ReadAllText("./public.dat"));
byte[] cipherText = Encrypt(txt1.Text, restoredPublicKey);
txt2.Text = Convert.ToBase64String(cipherText);
}
/// <summary>
/// 還原公鑰
/// </summary>
/// <param name="base64PublicKey"></param>
/// <returns></returns>
private ECPublicKeyParameters RestorePublicKeyFromBase64(string base64PublicKey)
{
// 將Base64格式的公鑰字符串轉換為字節數組
byte[] publicKeyBytes = Convert.FromBase64String(base64PublicKey);
// 獲取SM2曲線參數,這里使用sm2p256v1曲線
X9ECParameters sm2Params = GMNamedCurves.GetByName("sm2p256v1");
// 創建ECDomainParameters對象,包含曲線的一些基本參數,如曲線、生成元G、階N和系數H
var ecParams = new ECDomainParameters(sm2Params.Curve, sm2Params.G, sm2Params.N, sm2Params.H);
// 使用曲線參數解碼公鑰字節數組,將其轉換為ECPoint
var q = sm2Params.Curve.DecodePoint(publicKeyBytes);
// 根據解碼后的ECPoint和ECDomainParameters創建ECPublicKeyParameters對象
return new ECPublicKeyParameters(q, ecParams);
}
/// <summary>
/// 加密
/// </summary>
/// <param name="plainText"></param>
/// <param name="publicKey"></param>
/// <returns></returns>
private byte[] Encrypt(string plainText, ECPublicKeyParameters publicKey)
{
var sm2Engine = new SM2Engine();
sm2Engine.Init(true, new ParametersWithRandom(publicKey, new SecureRandom()));
byte[] plainBytes = System.Text.Encoding.UTF8.GetBytes(plainText);
return sm2Engine.ProcessBlock(plainBytes, 0, plainBytes.Length);
}
private void btnDecrypt_Click(object sender, EventArgs e)
{
var restoredPrivateKey = RestorePrivateKeyFromBase64(File.ReadAllText("./private.dat"));
string decryptedText = Decrypt(txt2.Text, restoredPrivateKey);
txt3.Text = decryptedText;
}
/// <summary>
/// 解密
/// </summary>
/// <param name="base64CipherText"></param>
/// <param name="privateKey"></param>
/// <returns></returns>
private static string Decrypt(string base64CipherText, ECPrivateKeyParameters privateKey)
{
var sm2Engine = new SM2Engine();
sm2Engine.Init(false, privateKey);
byte[] cipherBytes = Convert.FromBase64String(base64CipherText);
byte[] decryptedBytes = sm2Engine.ProcessBlock(cipherBytes, 0, cipherBytes.Length);
return System.Text.Encoding.UTF8.GetString(decryptedBytes);
}
/// <summary>
/// 還私公鑰
/// </summary>
/// <param name="base64PrivateKey"></param>
/// <returns></returns>
private ECPrivateKeyParameters RestorePrivateKeyFromBase64(string base64PrivateKey)
{
// 將Base64格式的私鑰字符串轉換為字節數組
byte[] privateKeyBytes = Convert.FromBase64String(base64PrivateKey);
// 使用BigInteger構造函數將字節數組轉換為無符號大整數,這將表示我們的私鑰
BigInteger d = new BigInteger(1, privateKeyBytes);
// 獲取SM2曲線參數,這里使用sm2p256v1曲線
X9ECParameters sm2Params = GMNamedCurves.GetByName("sm2p256v1");
// 創建ECDomainParameters對象,包含曲線的一些基本參數,如曲線、生成元G、階N和系數H
var ecParams = new ECDomainParameters(sm2Params.Curve, sm2Params.G, sm2Params.N, sm2Params.H);
// 根據無符號大整數和ECDomainParameters創建ECPrivateKeyParameters對象,表示私鑰
return new ECPrivateKeyParameters(d, ecParams);
}
}
}
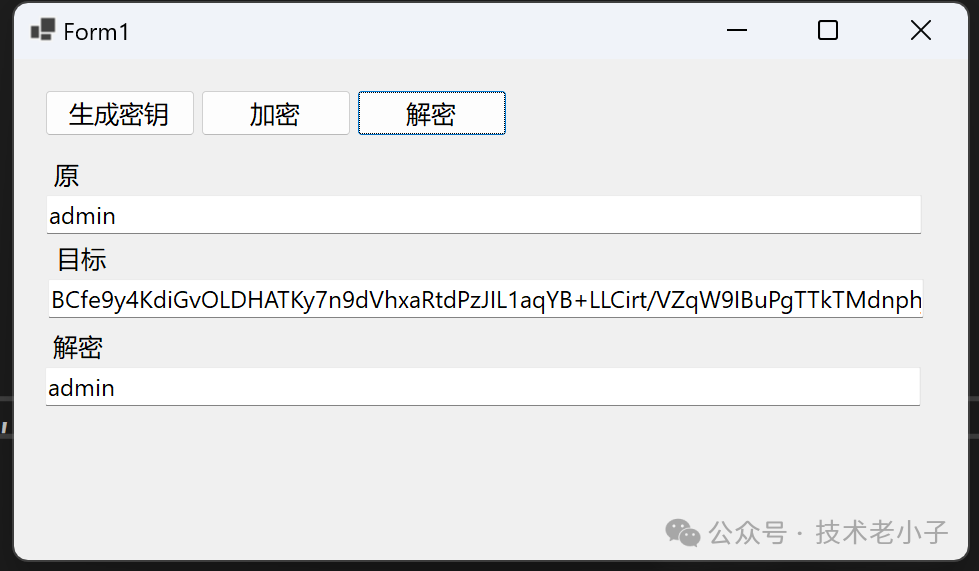
代碼說明
生成密鑰對:使用GenerateSM2KeyPair
方法生成SM2密鑰對。
加密:用公鑰加密明文,返回密文。
解密:用私鑰解密密文,返回明文。
小結
本文介紹了SM2算法的基本特點、應用場景,并提供了一個完整的C#示例代碼。通過BouncyCastle庫,開發者可以方便地在C#應用程序中實現SM2加密和解密操作。
SM2作為中國國家密碼標準,在確保信息安全傳輸方面起到了至關重要的作用。在未來的應用中,SM2有望在更多領域中發揮更大的作用。
該文章在 2024/7/22 12:18:38 編輯過