近期,我在致力于打造自己的小程序產品時,迎來了一項關鍵性的進展——微信相關授權流程的完整實現。從用戶登錄到權限獲取,我們細致入微地梳理并實現了每一項授權機制,確保了用戶體驗的流暢與安全。
微信小程序授權
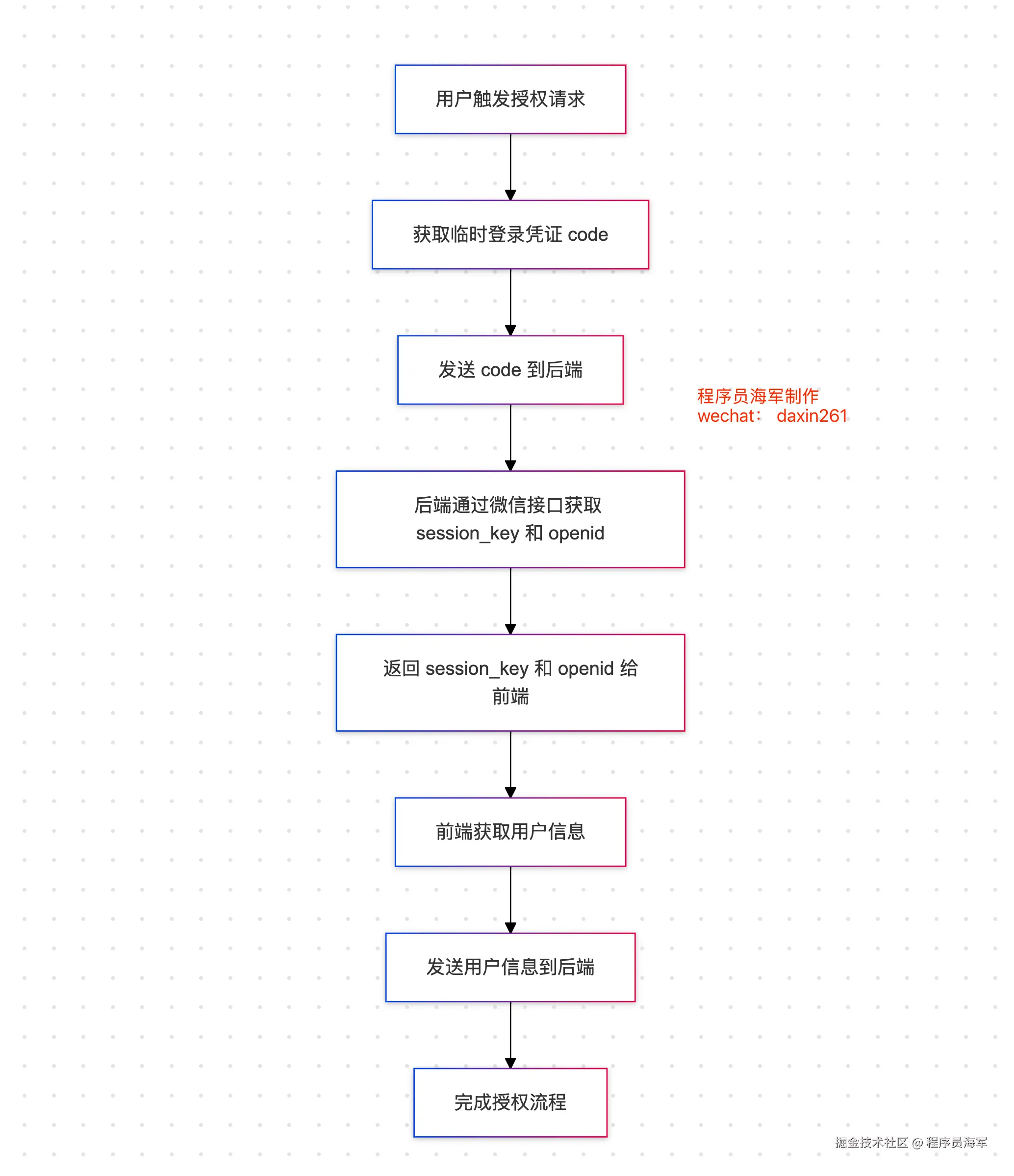
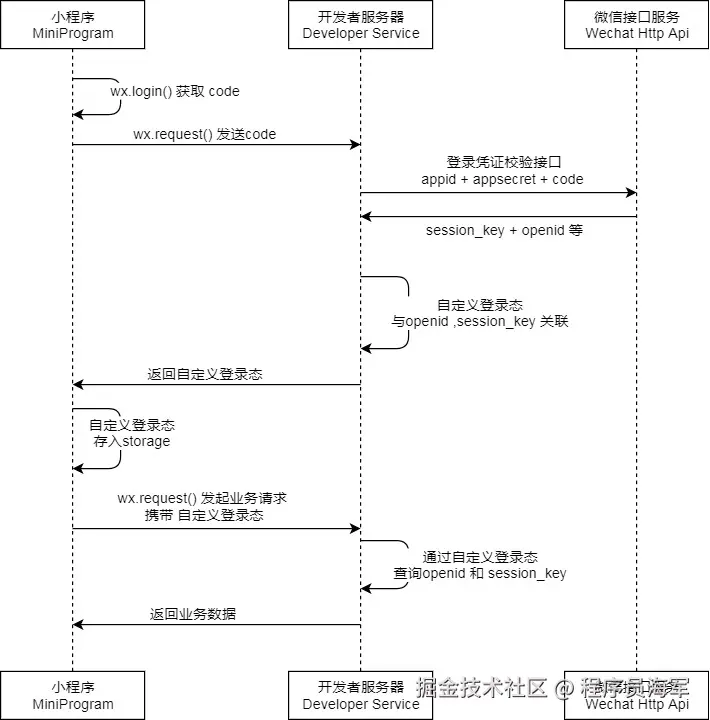
授權流程:
- 用戶在小程序中點擊登錄按鈕,觸發
wx.login()
獲取 code
。 - 小程序將
code
發送到后端服務器。 - 后端通過微信接口
jscode2session
使用 code
獲取 session_key
和 openid
。 - 后端返回
session_key
和 openid
給前端。 - 前端獲取
session_key
和 openid
,使用 wx.getUserProfile()
獲取用戶信息(如昵稱、頭像等)。 - 如果需要,可以將用戶信息(如昵稱、頭像等)發送到后端進行存儲或處理。
wx.login({
success: function(res) {
if (res.code) {
// 將 code 發送到服務器
wx.request({
url: 'https://localhost:8080/api/login',
method: 'POST',
data: {
code: res.code
},
success: function(response) {
// 處理服務器返回的數據
console.log(response.data);
}
});
}
}
});
下面是Nest 偽代碼實現
import { Injectable } from '@nestjs/common';
import { HttpService } from '@nestjs/axios';
import { firstValueFrom } from 'rxjs';
interface MiniProgramLoginResponse {
openid: string;
session_key: string;
unionid?: string;
errcode?: number;
errmsg?: string;
}
@Injectable()
export class MiniProgramAuthService {
constructor(private readonly httpService: HttpService) {}
async login(code: string): Promise<MiniProgramLoginResponse> {
const url = 'https://api.weixin.qq.com/sns/jscode2session';
try {
const response = await firstValueFrom(
this.httpService.get(url, {
params: {
appid: process.env.MINIPROGRAM_APPID,
secret: process.env.MINIPROGRAM_APPSECRET,
js_code: code,
grant_type: 'authorization_code'
}
})
);
return response.data;
} catch (error) {
throw new Error('小程序登錄失敗');
}
}
async decryptUserInfo(sessionKey: string, encryptedData: string, iv: string) {
}
}
微信網頁授權(OAuth 2.0)
網頁授權是通過微信官方提供的OAuth2.0認證方式,使第三方網站或應用能夠獲取用戶基本信息,實現用戶身份識別。
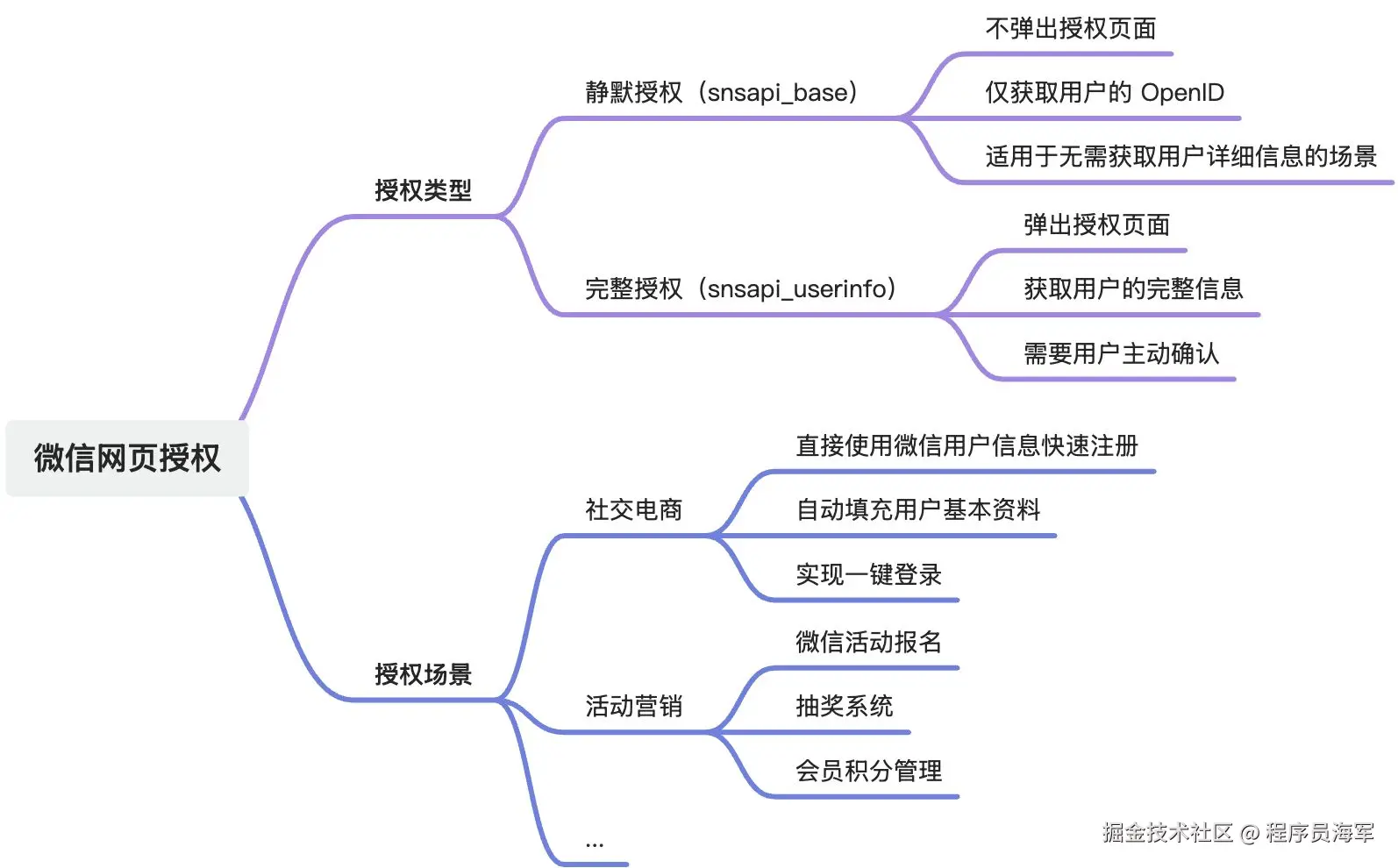
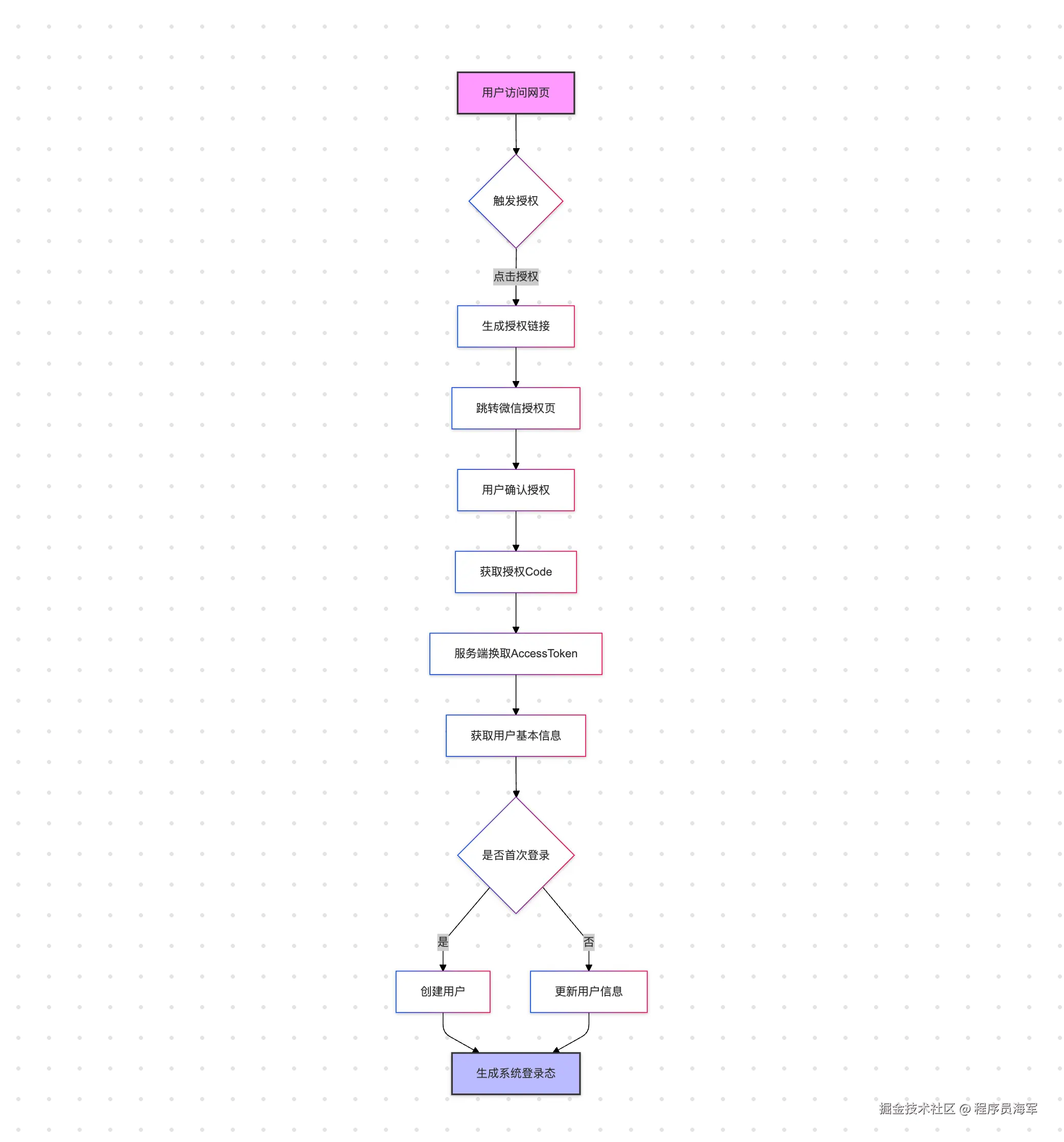
授權流程
- 發起授權
- 用戶點擊登錄/授權按鈕
- 生成授權鏈接
- 跳轉至微信授權頁面
- 用戶確認
- 換取 Access Token
- 服務端使用
code
換取 access_token
- 獲取用戶的
openid
和 access_token
- 獲取用戶信息
- 使用
access_token
和 openid
- 調用微信接口獲取用戶詳細信息
- 系統內部處理
import { Injectable } from '@nestjs/common';
import { HttpService } from '@nestjs/axios';
import { firstValueFrom } from 'rxjs';
interface WechatUserInfo {
openid: string;
nickname: string;
sex: number;
province: string;
city: string;
country: string;
headimgurl: string;
privilege: string[];
unionid?: string;
}
@Injectable()
export class WebAuthService {
constructor(private readonly httpService: HttpService) {}
generateAuthUrl(redirectUri: string, scope: 'snsapi_base' | 'snsapi_userinfo' = 'snsapi_userinfo') {
const baseUrl = 'https://open.weixin.qq.com/connect/oauth2/authorize';
const params = new URLSearchParams({
appid: process.env.WECHAT_APPID,
redirect_uri: redirectUri,
response_type: 'code',
scope: scope,
state: 'STATE#wechat_redirect'
});
return `${baseUrl}?${params}#wechat_redirect`;
}
async getAccessToken(code: string) {
const url = 'https://api.weixin.qq.com/sns/oauth2/access_token';
try {
const response = await firstValueFrom(
this.httpService.get(url, {
params: {
appid: process.env.WECHAT_APPID,
secret: process.env.WECHAT_APPSECRET,
code: code,
grant_type: 'authorization_code'
}
})
);
return response.data;
} catch (error) {
throw new Error('獲取 Access Token 失敗');
}
}
async getUserInfo(accessToken: string, openid: string): Promise<WechatUserInfo> {
const url = 'https://api.weixin.qq.com/sns/userinfo';
try {
const response = await firstValueFrom(
this.httpService.get(url, {
params: {
access_token: accessToken,
openid: openid,
lang: 'zh_CN'
}
})
);
return response.data;
} catch (error) {
throw new Error('獲取用戶信息失敗');
}
}
}
微信開放平臺授權
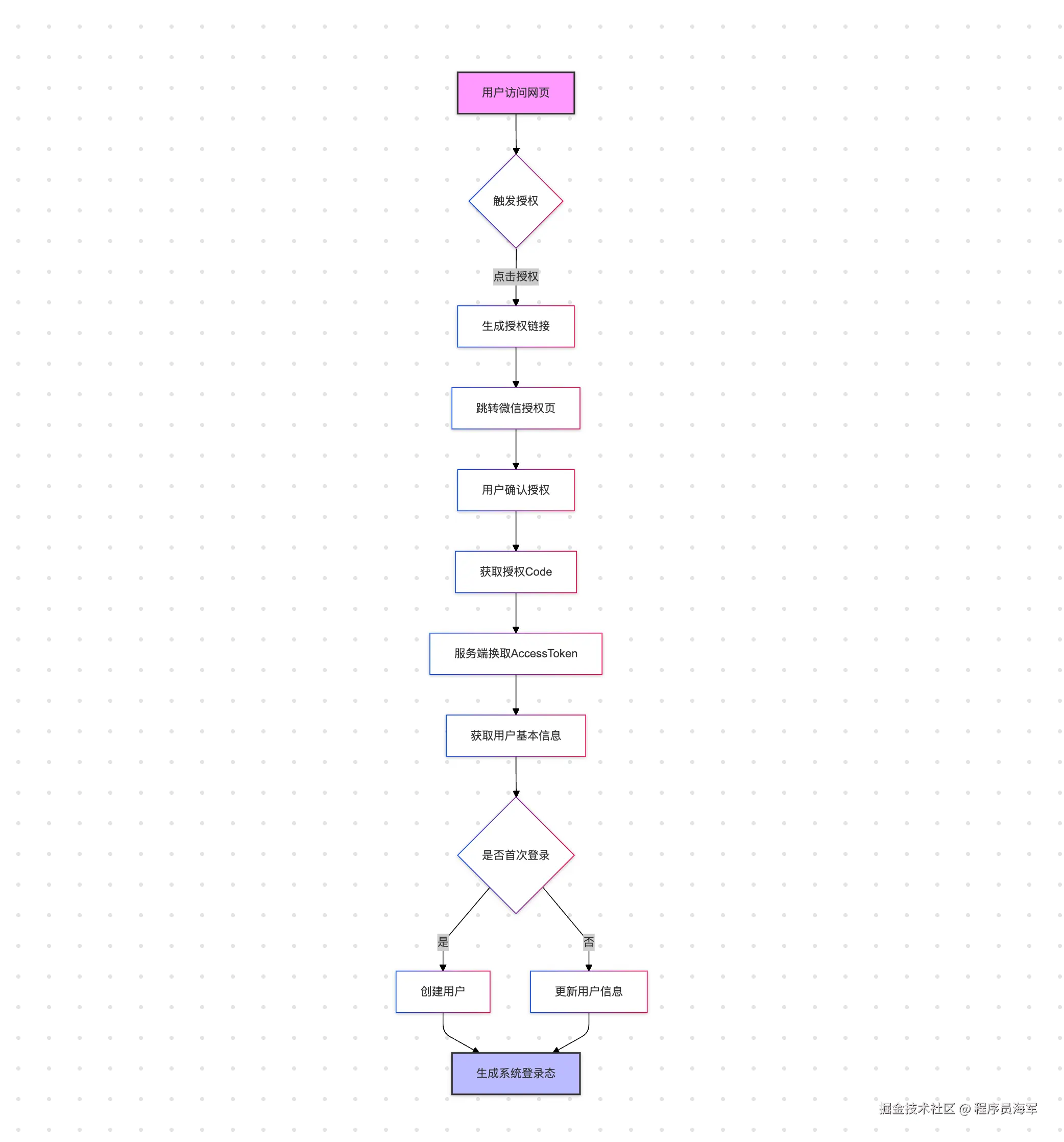
特點:
- 適用于第三方應用
- 支持移動應用、網站應用等
- 需要開發者資質認證
import { Injectable } from '@nestjs/common';
import { HttpService } from '@nestjs/axios';
import { firstValueFrom } from 'rxjs';
interface OpenPlatformAuthResponse {
access_token: string;
expires_in: number;
refresh_token: string;
openid: string;
scope: string;
unionid: string;
}
@Injectable()
export class OpenPlatformAuthService {
constructor(private readonly httpService: HttpService) {}
async getAccessToken(code: string): Promise<OpenPlatformAuthResponse> {
const url = 'https://api.weixin.qq.com/sns/oauth2/access_token';
try {
const response = await firstValueFrom(
this.httpService.get(url, {
params: {
appid: process.env.OPEN_PLATFORM_APPID,
secret: process.env.OPEN_PLATFORM_APPSECRET,
code: code,
grant_type: 'authorization_code'
}
})
);
return response.data;
} catch (error) {
throw new Error('開放平臺授權失敗');
}
}
async refreshAccessToken(refreshToken: string) {
const url = 'https://api.weixin.qq.com/sns/oauth2/refresh_token';
try {
const response = await firstValueFrom(
this.httpService.get(url, {
params: {
appid: process.env.OPEN_PLATFORM_APPID,
grant_type: 'refresh_token',
refresh_token: refreshToken
}
})
);
return response.data;
} catch (error) {
throw new Error('刷新 Token 失敗');
}
}
}
企業微信授權
企業微信授權是針對企業內部應用和員工的身份認證機制,提供更嚴格和精細的權限控制。
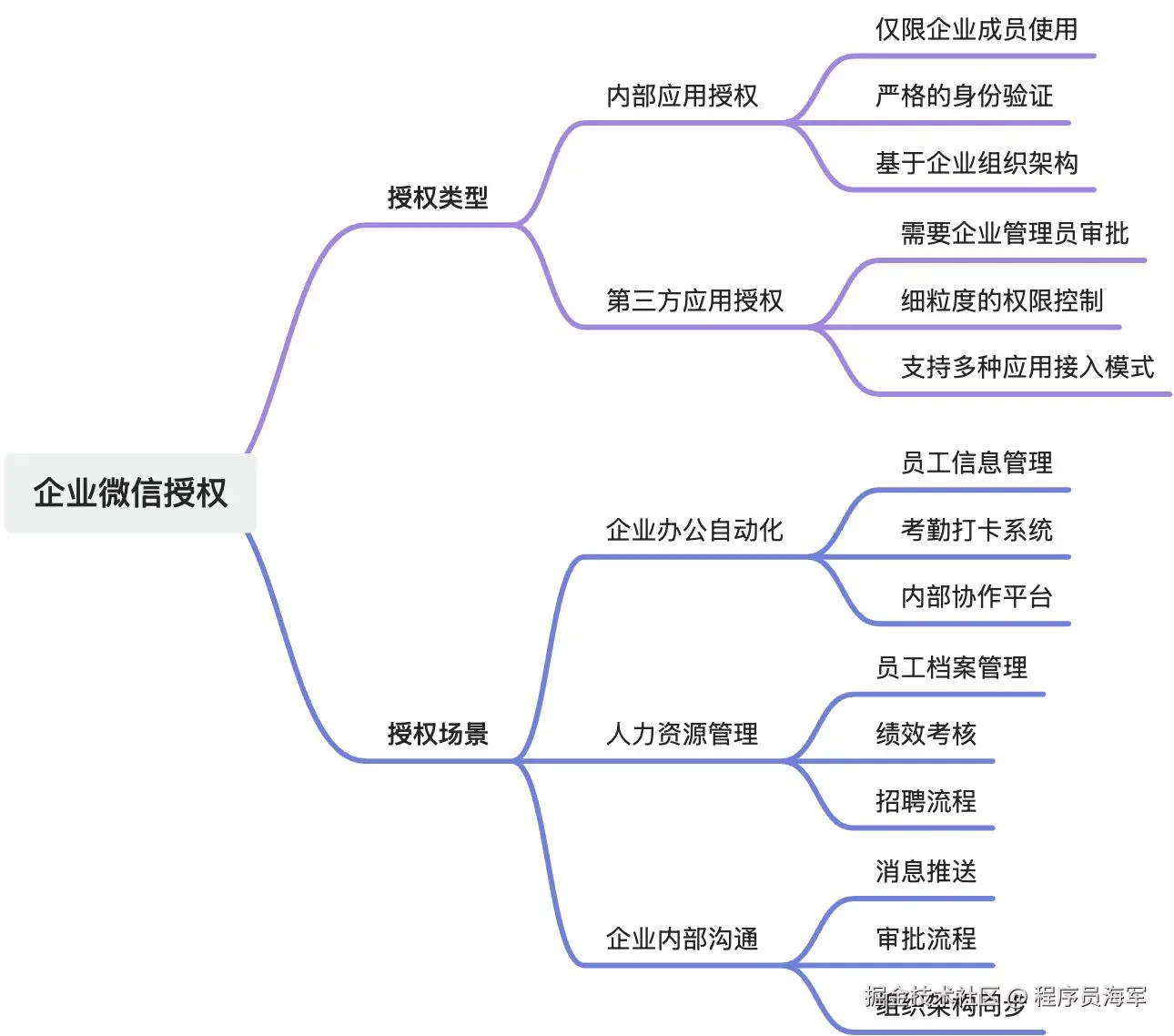
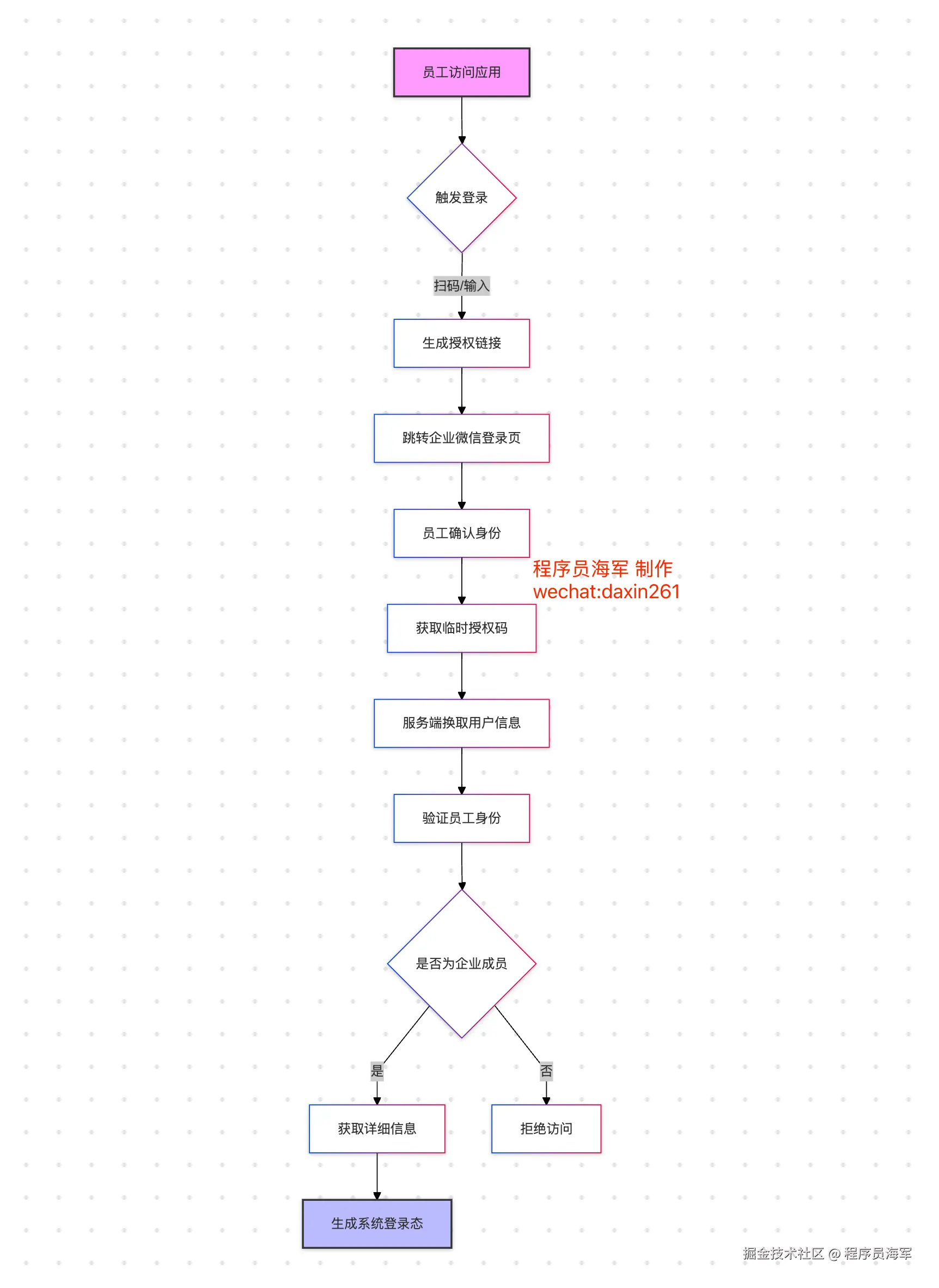
特點:
授權流程
- 發起授權
- 員工訪問企業內部應用
- 觸發登錄機制(掃碼/輸入)
- 生成企業微信授權鏈接
- 身份驗證
- 換取用戶信息
- 服務端使用
code
換取用戶標識 - 獲取
userid
- 調用接口獲取用戶詳細信息
- 系統內部處理
import { Injectable } from '@nestjs/common';
import { HttpService } from '@nestjs/axios';
import { firstValueFrom } from 'rxjs';
interface EnterpriseWechatAuthResponse {
access_token: string;
expires_in: number;
user_ticket?: string;
user_info?: {
userid: string;
name: string;
department: number[];
};
}
@Injectable()
export class EnterpriseWechatAuthService {
constructor(private readonly httpService: HttpService) {}
async getUserInfo(code: string): Promise<EnterpriseWechatAuthResponse> {
const tokenUrl = 'https://qyapi.weixin.qq.com/cgi-bin/gettoken';
const userInfoUrl = 'https://qyapi.weixin.qq.com/cgi-bin/user/getuserinfo';
const tokenResponse = await firstValueFrom(
this.httpService.get(tokenUrl, {
params: {
corpid: process.env.ENTERPRISE_CORPID,
corpsecret: process.env.ENTERPRISE_CORPSECRET
}
})
);
const accessToken = tokenResponse.data.access_token;
const userInfoResponse = await firstValueFrom(
this.httpService.get(userInfoUrl, {
params: {
access_token: accessToken,
code: code
}
})
);
return userInfoResponse.data;
}
}
各個平臺授權小結
授權類型 | 個人是否可用 | 是否收費 | 主要適用場景 |
---|
網頁授權 | 是 | 免費 | 網站、H5應用 |
小程序授權 | 是 | 免費 | 小程序登錄、開放平臺 |
公眾號授權 | 部分可用 | 免費+增值服務 | 公眾號相關應用(服務號、訂閱號) |
企業微信 | 否 | 有費用 | 企業內部協作 |
?轉自https://www.cnblogs.com/HaiJun-Aion/p/18609286
該文章在 2024/12/17 15:49:13 編輯過